Java parameters
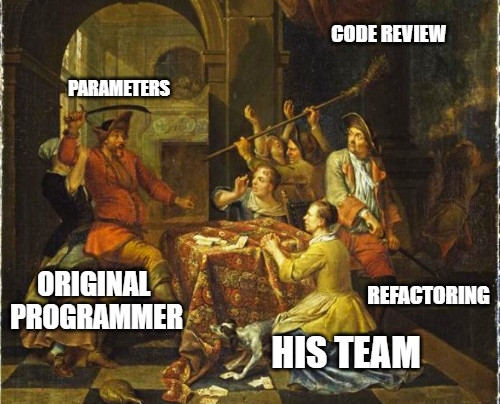
How many parameters should a method have?
The answer is the fewest possible.
Maximum three.
Preferably zero.
Arguments are bad.
Each argument brings more chaos into your function.
Each argument increases the complexity of your method.
The more arguments your method has the harder it is to use.
Especially dangerous are same type arguments.
The more same type arguments your method has, the easiest it is to confuse them and create bugs.
Another category of parameters you especially should try to stay away from, are parameters passed by reference, that change state inside your function.
You risk modifying the state of objects where no one expects you to modify it.
That is, far away from where it should be modified and where the state is used.
If you can’t avoid changing the state of parameters, at least make it clear you are doing so.
You can do this by returning that parameter.
If you can’t return it, you can show this change with the name of the function.
If this is also not possible, as a last resort you can use comments.
So it is best to avoid changing the state of your parameters.
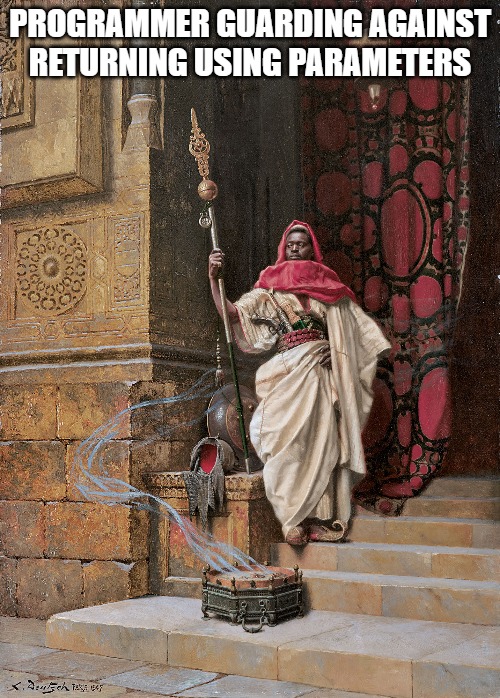
Changing state is important.
You should make it as clear as possible when you are doing it.
Your code should scream:
HEY! I CHANGE THE STATE OF THIS OBJECT INSIDE MY FUNCTION! BE CAREFUL HERE!
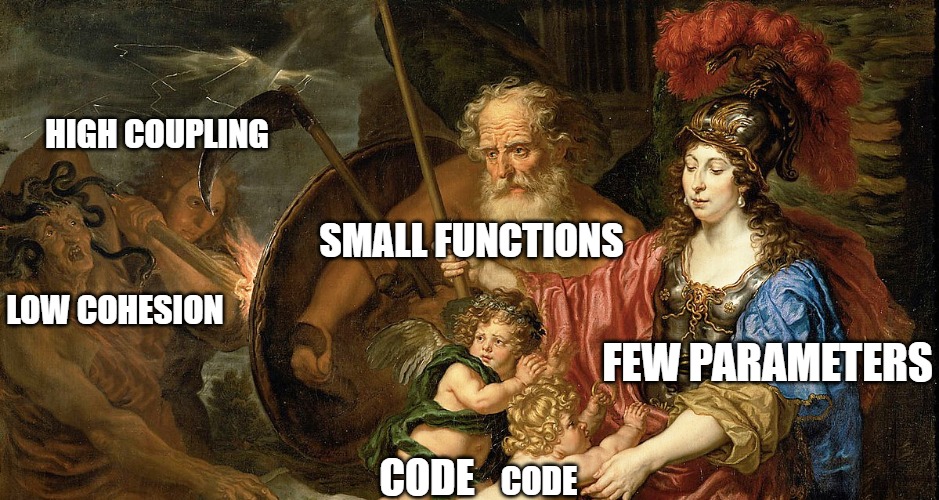
Parameters also couple your method to the outside.
The more parameters your method has, the more coupled it is.
What if I need many parameters?
I need to pass five different strings.
There’s no way around it.
Create a new class then.
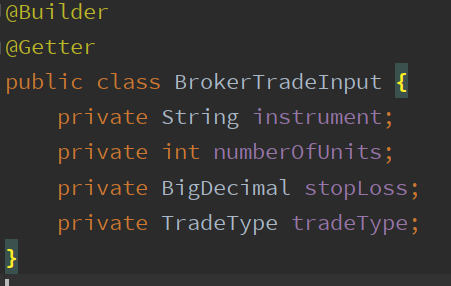
The fact that these variables are sent together means they have enough cohesion to be together in a class.
If you can’t find any good name for what they represent together, just add an input at the end of the function name.
I like to make these types of objects immutable, so I only give them getters and a builder from Lombok.
That way you can create the object but nobody will try to set its contents.
He can still change it do it, but you send a clue that he shouldn’t.
You can make it an inner class or put it in the same package, or create a package just for these types of parameter classes, if they don’t seem to belong anywhere else.
All of the things that I said until now also apply to constructors.
It is common for constructors to take many arguments.
In this case you can either create a parameters class or use a builder.
Never use Boolean arguments.
If you use Boolean parameters, it means you are doing more than one thing in your method.
Also it’s hard to figure out what Boolean arguments do.
Intellij actually helps now by showing the parameters name at the calling place.
Instead of using Booleans, create two methods, one for true and one for false.
Null arguments are just like Booleans so the same rules apply: create two functions, one for null and one for value.
Only expect to receive null when the users of your method are outside of your team.
Checking all arguments for null is called defensive programming.
It should not happen inside a team.
Only for public APIs.
This article is part of the “Parameters” episode from my Clean Code course.
You can watch the Clean Code courses here:
– Watch Clean code with Java examples course on Udemy
– Watch Clean code with PHP examples course on Udemy
– Watch this Clean code with Java examples – Basics for free here AND get 2 FREE months of skillshare.com Premium